- Ontriggerexit How To Test For Player Tag Unity
- Ontriggerstay Vs Ontriggerenter Unity
- Unity Ontriggerstay
When OnTriggerExit occurs, the GameObjects are deactivated and components disabled. This component is useful to enable components such as Bark On Idle only when the player enters a trigger collider that represents proximity to the barker. You can also configure UnityEvents to run on enter and exit. Trigger Event, Collision Event, Timed Event.
- OnTriggerEnter( ), OnTriggerStay( ), and OnTriggerExit( ) These methods are only used with a game object that a collider attached. And the collider must be set as a trigger. They are called when a collider interacts with the game object. You can check this article for more information about Trigger and Collision Methods.
- Similarly to the colliders, OnTriggerStay method will be called each frame as long as an object will be inside a trigger. And at the end, when an object will leave the trigger, Unity will invoke OnTriggerExit method. Fun fact is that that these functions will be invoked on both objects – Trigger and object which entered the Trigger.
In your games, you will often need to detect collisions between objects. This can be related to NPCs or objects that you will collect or interact with. For this purpose, you can use (and monitor) colliders. However, the way you manage collisions, and the methods to be called in this case, will highly depend on the type of colliders used (for example, colliders or triggers) as well as the object from which you want to detect the collision (for example, simple objects or character controllers).
This being said, you may want to be able to detect the presence of objects located far away from the player or the NPC. In this particular case, ray-casting may make more sense than collision or trigger detection.
So this tutorial will explain in great details how you can detect objects using a wide range of techniques such as colliders, triggers and ray-casting.
In your games, you will often need to detect collisions between objects. This can be related to NPCs or objects that you will collect or interact with. For this purpose, you can use (and monitor) colliders. However, the way you manage collisions, and the methods to be called in this case, will highly depend on the type of colliders used (for example, colliders or triggers) as well as the object from which you want to detect the collision (for example, simple objects or character controllers).
So, let’s start with the most likely scenario where you have to collect objects using a first-person controller. You could create a script, add the following code to it, and drag and drop the script on the FPSController object.
In the previous code, we detect collision between the player (when it is in in movement) and other colliders in the scene. This method returns a ControllerColliderHit object that provides information about the collision, including the collider involved and its associated GameObject.
Next, we could try to detect collision between a third-person controller and other objects. So you could create a script, add the following code to it, and drag and drop the script on the ThirdPersonController object. This code will work because the methods OnCollisionEnter and OnCollisionExit require a collision between a rigid body and a collider and since the ThirdPersonController object includes a rigid body then these conditions are fulfilled.
In the previous code, we use both the methods OnCollisionEnter and OnCollisionExit, and, each time, we display the name of the object that is (or was) colliding with the character.
In addition to the two previous examples, when you want to detect collision between your character and other objects, you might also want to detect when your character is entering a specific zone. For example, it may be the case that an alarm should be raised when the player enters a specific room, or maybe the player’s energy should replenish after entering a “healthy” area. In both cases, you don’t need to detect collisions. Instead, you just need to define an area based on a spherical, cylindrical or cubical primitive and call a specific function when an object enters this area.
To define areas that act as triggers, you can use simple primitives (for example a cube, a sphere or a cylinder). When you create a primitive in Unity, it will include a collider by default, and this collider can be set to a normal mode (that is, the collider mode) or to a trigger mode. This can be done using the Inspector by enabling or disabling the attribute called IsTrigger for the Collider component (for example, the BoxCollider component if the primitive is a box).
In our case, we would need to:
- Set the parameter IsTriger to true.
- Deactivate the Renderer for this box so that the trigger area is not visible in the game.
- Add the following code to a script attached to either a First- or a Third-PersonController as follows.
In the previous code, we use both the methods OnTriggerEnter and OnTriggerExit, and we then display the name of the objects that are used to define the trigger area. In both cases, no collision will be detected and the player will be able to walk through the other objects, as these objects will only be acting as triggers. Note that this script would also work if it was added to the primitive that defines the trigger area.
Ray-casting implies casting a virtual ray in a specific direction and testing whether an object “collides” with the ray. When you design this ray, its origin may differ; sometimes, for example in FPS games, you may want it to originate from the middle of the screen. In other cases, you may prefer the ray to be created just ahead of an NPC so that it can detect objects ahead. So, we will see how each of these can be employed.
This technique is particularly useful when using First-Person Controllers so that the raycast points exactly in the same direction as where the player looks. In this case, we could create a script that is attached to the object FirstPersonCharacter (which is a child of the object FPSController used for a First-Person Controller). This is very important because the script will be linked to the latter. If you were to add this script to the object FPSController instead, an error would occur because this object does not have a camera component, and the script will still need to use this camera.
The following script illustrates how this could be done.
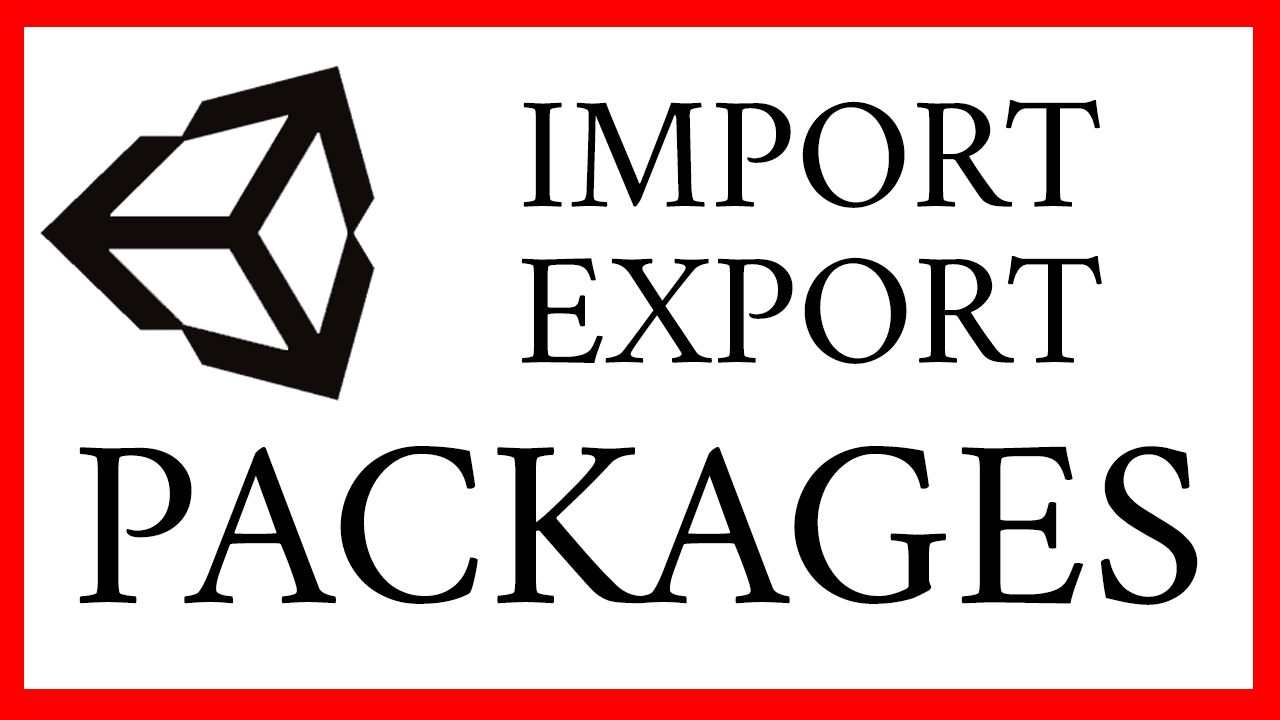
In the previous code, we do the following:
- We initialize our ray defined earlier. This ray will be originating from the camera used for the First-Person Controller, from the center of the screen, which is defined by the x and y coordinates width/2 (that is, half of the screen’s width) and Screen.height/2 (that is, half of the screen’s height). The z coordinate is ignored since we consider the screen as two-dimensional space. So at this stage, we know where the ray will start and, by default, it will point outwards.
- On the next line, we use the static method DrawRay and specify three parameters: the origin of the ray, its direction, and its color. By using the syntax origin we will start the ray from the middle of the screen. By using the syntax rayFromPlayer.direction*100, we specify that the ray’s length is 100 meters. This ray can only be seen in the Scene view, but not the Game view.
- We cast a ray using the keyword RayCast. The method RayCast takes three parameters: the ray (rayFromPlayer), an object where the information linked to the collision between the ray and another collider is stored (hit), and the length of the ray (100). The keyword out is used so that the information returned about the collision is easily accessible (this is comparable to a type conversion or casting).
- If this ray hits an object (i.e., the collider from an object), we print a message that displays the name of this object. To obtain this name, we access the collider involved in the collision, then the corresponding GameObject using the syntax collider.gameObject.

The method Debug.DrawRay will create a ray that we can see in the scene view and that can be used for debugging purposes to check that a ray effectively points in the right direction. However, Debug.DrawRay does not detect collisions with objects. So while it is useful to check the direction of a particular ray in the Scene view, this ray needs to be combined to a different method to be able to detect collisions and one of these methods is called Physics.Raycast.
Ontriggerexit How To Test For Player Tag Unity
There may be cases when you want to cast a ray from an object. For example, you might want to equip NPCs with the ability to see and detect objects ahead. In this case, you could create a ray that originates just a few centimeters ahead of the NPC and that is cast forward.
The only difference with the previous example would be the creation of the ray; so we would, in this case, replace this line.
… with this line …
Ontriggerstay Vs Ontriggerenter Unity
In the previous code we create a ray that starts 1.5 meters ahead of the player and that is pointing forward.
If you’d like to know more about C# coding, this resource will help you to write better, and faster code.
Unity Ontriggerstay
Related Articles:
